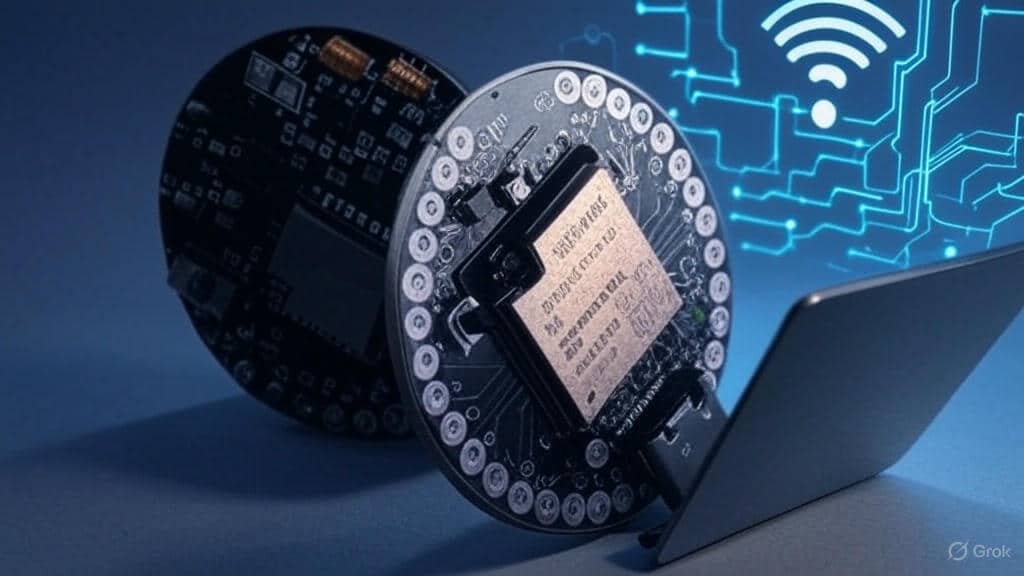
Check out our latest products
In the era of interconnected devices, securing IoT systems is paramount to protect sensitive data from unauthorized access. This project demonstrates an IoT Security System leveraging AES (Advanced Encryption Standard) encryption to transform plain-text data into a “secret code” that only authorized users can decipher.
By integrating AES with an IndusBoard Coin, we ensure that data transmitted over Wi-Fi, such as sensor readings or user messages, remains confidential and tamper-proof. The system aligns with the IndusBoard Coin Security System concept, emphasizing robust encryption for IoT applications.
AES is a symmetric encryption algorithm widely used for its efficiency and strength. Here, we use it to encrypt data on the Coin board, which supports hardware-accelerated AES via its cryptographic engine. This project evolves in two parts:
- A standalone encryption demo using AES-256.
- An IoT sender-receiver system with a web UI for encrypting and decrypting messages in AP/STA mode.
Supported on any board and CHIP that features built-in AES hardware acceleration. The IndusBoard Coin Security System could theoretically integrate with IoT, using AES alongside secure elements like the ATECC608A for key storage, though this project focuses on software-based AES for simplicity.
AES (Advanced Encryption Standard) is a symmetric encryption algorithm established by the U.S. National Institute of Standards and Technology (NIST) in 2001. It is widely used to secure data in applications ranging from banking to IoT devices due to its speed, efficiency, and robust security. AES operates on fixed-size blocks of data (16 bytes or 128 bits) and supports key sizes of 128, 192, or 256 bits—here, we use AES-128 and AES-256 variants.

How AES Works
- Key Expansion: The encryption key (e.g. 32 bytes for AES-256) is expanded into a set of round keys using a key schedule. Each round key is used in subsequent transformation steps.
- Encryption Process:
- SubBytes: Each byte of the 16-byte block is substituted using a predefined S-box (Substitution box), adding non-linearity.
- ShiftRows: Rows of the block are shifted cyclically to diffuse the data.
- MixColumns: Columns are mixed using a mathematical transformation to further scramble the data.
- AddRoundKey: The block is XORed with a round key. This process repeats for multiple rounds (10 for AES-128, 14 for AES-256).
- Decryption: The inverse operations (InvSubBytes, InvShiftRows, etc.) are applied with the same key to recover the original data.
- Modes: AES can operate in modes like ECB (Electronic Codebook) or CBC (Cipher Block Chaining). ECB encrypts each block independently, while CBC uses an Initialization Vector (IV) to chain blocks, enhancing security.
Bill of Materials
Item | Description | Quantity |
---|---|---|
Board with AES Support and has a built-in secure engine (IndusBoard Coin) | IoT Board with AES encryption system | 1 |
Wi-Fi Network | For testing | 1 |
Micro USB Cable | For programming and power | 1 |
Part 1: Standalone AES-256 Encryption Demo
Code Explanation
The first code demonstrates AES-256 encryption and decryption using the mbedtls library.
Here in code, the inbuilt AES encryption is called, then a 256-bit AES encryption KEY is inserted in the code array. Next, it has a default message that you can change for encryption. Now, upload the code.
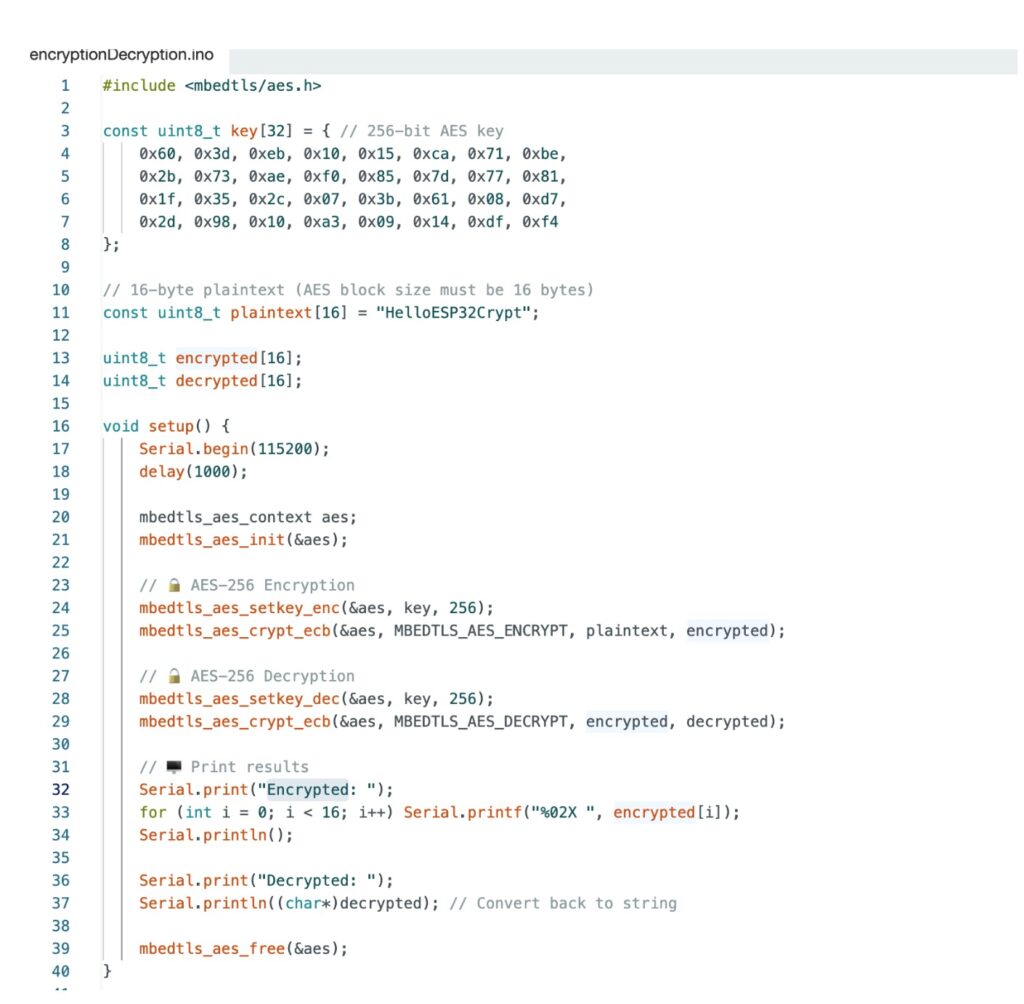
Testing AES-256 Encryption and Decryption
Upload the code to Coin via Arduino IDE. Open the Serial Monitor (115200 baud).

Then you can see the encryption and decryption process in serial.
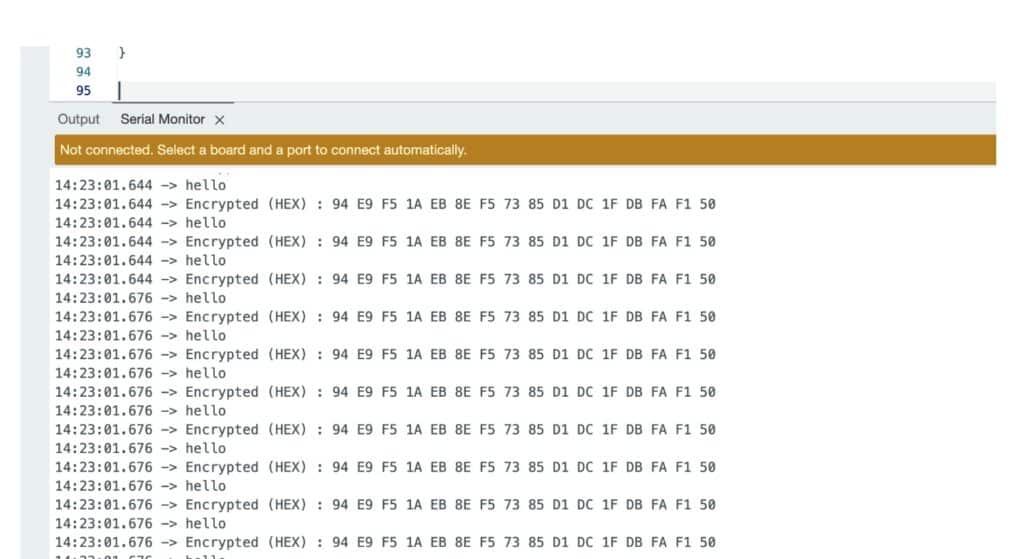
Part 2: IoT Encrypted Data Transmission with Web UI
Project Continuation: Sender-Receiver with Encryption
In our IoT system, AES encrypts messages sent from a web UI to a COIN sender, ensuring that intercepted Wi-Fi traffic appears as gibberish (e.g., 6EF23A…) without the key. The receiver decrypts it using the shared key, restoring the original message.
This protects confidentiality and aligns with the InusBoard Coin Security System concept by making data readable only to authorized parties.
This code modifies the previous sender-receiver system to use AES-128 with a web UI, allowing users to input messages, see encrypted output, and decrypt them on the Serial Monitor.
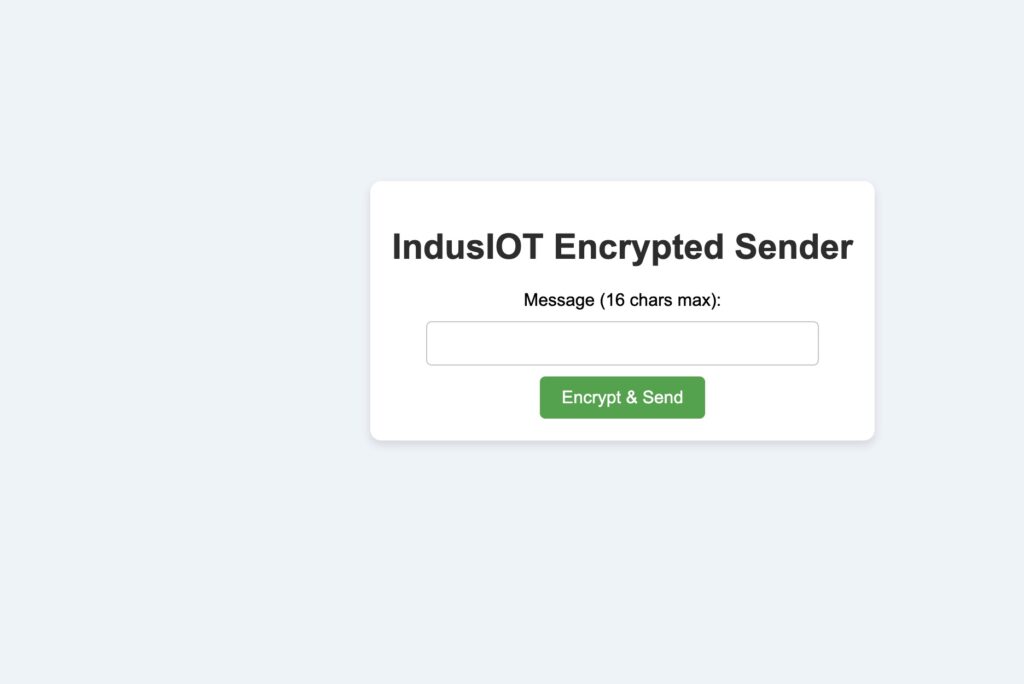
Code
- WiFi.h: Configures the ESP32 as a Wi-Fi Access Point (AP) to host the UI.
- WebServer.h: Manages HTTP requests and responses for the web interface.
- mbedtls/aes.h: Provides the AES encryption/decryption functions from the mbedTLS library, included in the ESP32 Arduino core.
ssid and password: Define the AP’s name and password (must be 8+ characters).
server(80): Creates a web server on port 80, the default HTTP port.
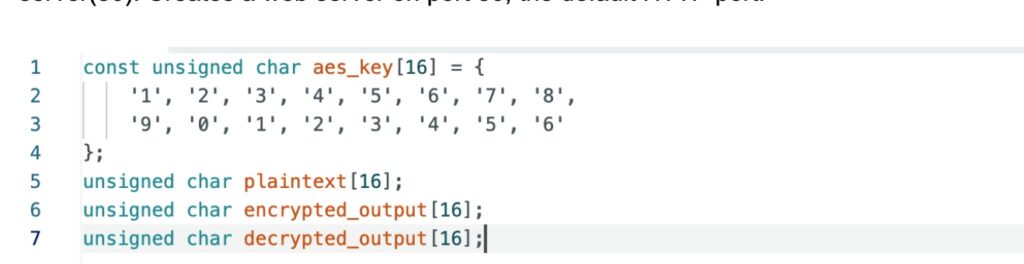
aes_key: A 16-byte (128-bit) key for AES-128, specified as individual characters to avoid string termination issues.
plaintext: Stores the 16-byte input message.
encrypted_output: Holds the encrypted ciphertext.
decrypted_output: Stores the decrypted result for verification.
Testing IoT Encrypted Data Transmission with Web UI
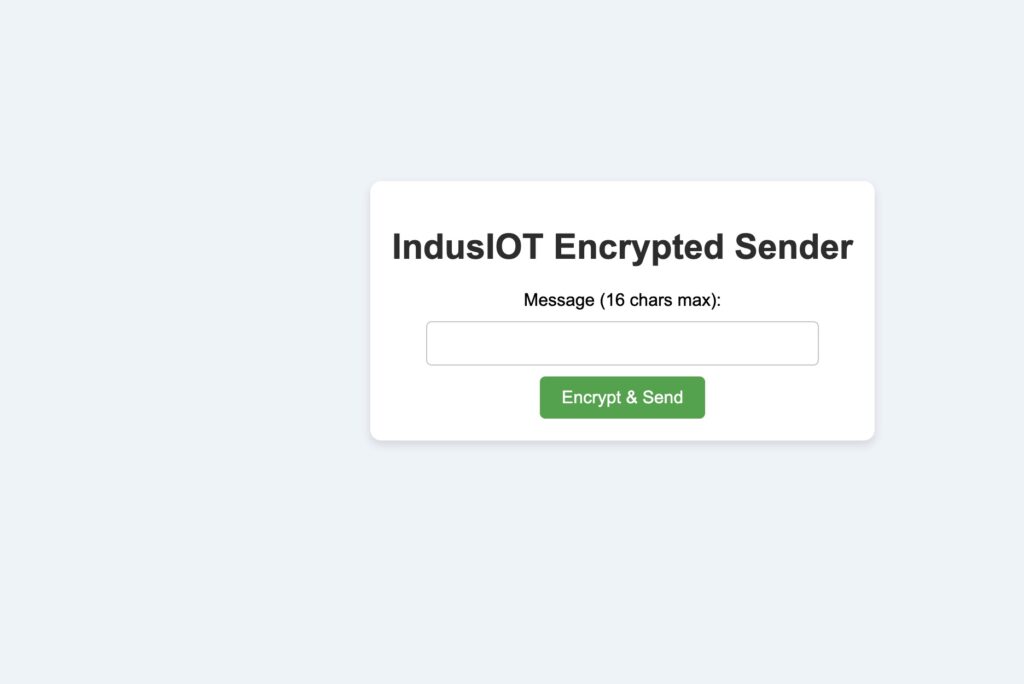

- Connect to the “IndusIoT security” Wi-Fi network (password: “12345678”).
- Open a browser and go to http://192.168.4.1.
- Enter a message (e.g., “Test1234”) and submit.
- Check the Serial Monitor (115200 baud) for encrypted output and decrypted message